Lab: GUIs, Factories, Screensavers
Problem Statement
It is often possible to create a number of shapes in a specific pattern algorithmically (i.e., using a loop and calculating the position, size, speed, direction, etc.). When combined with movement, this has the possibility of producing a very interesting effect, with very little effort. This is the basis of most screen savers.
Set Up
Accept the assignment and clone the repository. In Eclipse, you'll do this in the Git repository view. Import the project into Eclipse, as you have done a few times.
Screen Savers
For each of the screen savers below, you will create an appropriate
child class of the Factory
class and implement its
createMovers
that, given a Canvas
and an
integer as parameters, creates the given number of shapes within the
given canvas in a specific pattern. The current Canvas
class will work without modification because you will modify the
paint
and move
methods of a separate class
that extends Mover
.
- Racers in a Line
Create a
Racer
class that is a derived class ofMover
. ImplementRacer
'spaint
andmove
methods such that it draws itself like a rectangle and animates itself by moving straight across the screen from left to right at a random rate (i.e., each timemove
is called, its speed should be randomly set between 1 and 10). When it reaches the right side it should stop, like those racing games at the local carnival.Create a
RacerFactory
class that is a derived class ofFactory
. Implement thecreateMovers
method to create the given number ofRacer
s such that they are all the same size and are positioned in a line along the left side of the canvas and exactly fill its height. AllRacer
s, no matter how many will be created, should start at the same center x-coordinate, 0, and have the same width, 20. The rest of their attributes should be set so that the rectangles are spread evenly across the edge of the canvas. For example, given 10 racers to create and a canvas that is 100 pixels tall, they should each be 10 pixels high and positioned with their center y-coordinates at 5, 15, 25, 35, 45, 55, 65, 75, 85, and 95, respectively.Add a button for the
RacerFactory
in theMain
class.When run, it should appear like the rectangles are racing to the right side of the window.
Note that you may have to do some minor adjustments to make the racers stretch the height of the canvas. The racers may not exactly be touching, but they'll appear to be to the user.
- Walkers on a Diagonal
Create a
Walker
class that draws itself like a rectangle and animates itself by moving at a constant rate in a random direction (i.e., each timemove
is called, its angle should be changed randomly). The speed of theWalker
should be constant throughout its life.Create a
WalkerFactory
to create the given number ofWalker
s such that they are all the same size and are positioned in a line diagonally across the canvas with each touching the two adjacent rectangles at their corners. Their position and size should be set so that they are spread evenly across the canvas. For example, given 10 walkers to create and a canvas whose size is 100x200 pixels, you should create 10 walkers that are 10x20 pixels in size and centered at (5, 10), (15, 30), (25, 50), (35, 70), (45, 90), (55, 110), (65, 130), (75, 150), (85, 170), and (95, 190), respectively.The colors of the attractors should all be within one "class" of colors (e.g., all red, blues, or greens).
Add a button for the
WalkerFactory
in theMain
class.When run, it should appear like the rectangles are taking a random (i.e., drunken) walk.
During the race
At the end of the race.
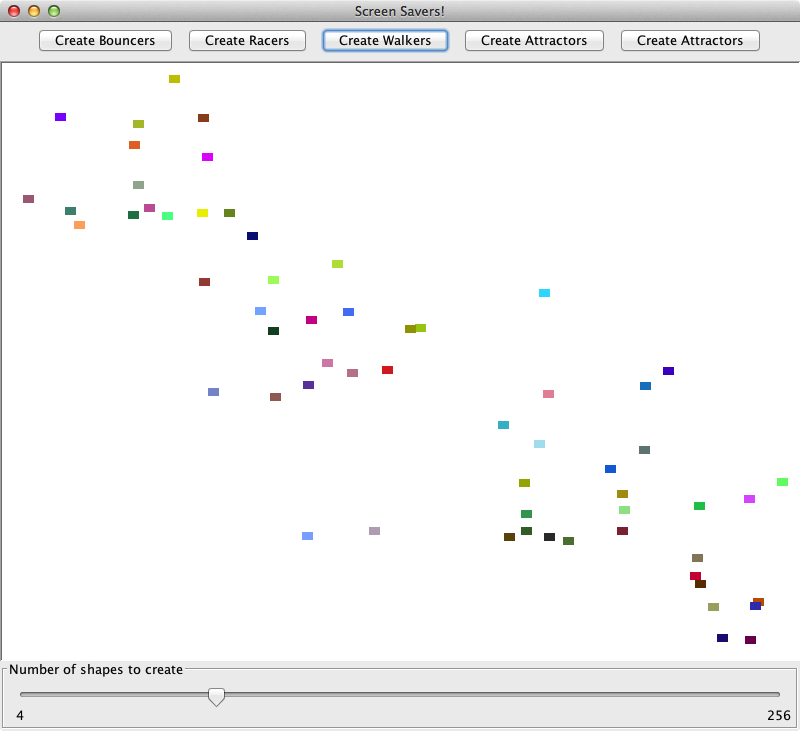
Learning More!
- Leaving a Trail
Change the super class,
Mover
, such that itpaint
s itself as a collection of connected line segments and animates itself by adding the current center of the shape to a collection of points (i.e., each timemove
is called, the center point is added to a collection). When drawn as a series of lines from the first point to the second point in the collection, from the second point to the third point, from the third point to the fourth point, and on and on until the last point in the collection, it will appear that the shape is leaving a trail behind marking where it has moved. To make the trail appear, each of the previous classes'paint
andmove
methods will need to be modified to call their correspondingsuper
method. Additionally,Mover
will need to declare and initialize an instance variable that is a collection of all the past points. - Interesting Circles
Implement an
AttractorFactory
class to create the given number ofAttractor
s such that they are drawn as circles, all the same size, and are positioned in a circular pattern with each touching the one beside it (i.e., tangent to each other) like the marks on a clock (if those marks were circles). In this case, their position and size should be set so that they are spread evenly around the perimeter of a circle whose diameter is the minimum of the width and height of the canvas. Recall that the formula for computing the circumference, or perimeter, of a circle is2 * Math.PI * radius
. For example, given 4 attractors to create and a canvas whose size is 100x200 pixels, you should create 4 attractors that are 100x100 pixels in size and centered at approximately (100, 0), (150, 50), (100, 100), and (50, 50), respectively.The colors of the attractors should all be within one "class" of colors (e.g., all red, blues, or greens).
Modify the
Attractor
such that it draws itself as a circle and animates itself in some "interesting" way. Options include orbiting, attracting towards the center as if by gravity, swarm effects, or simulating dynamical systems.Add a button for the
AttractorFactory
in theMain
class.
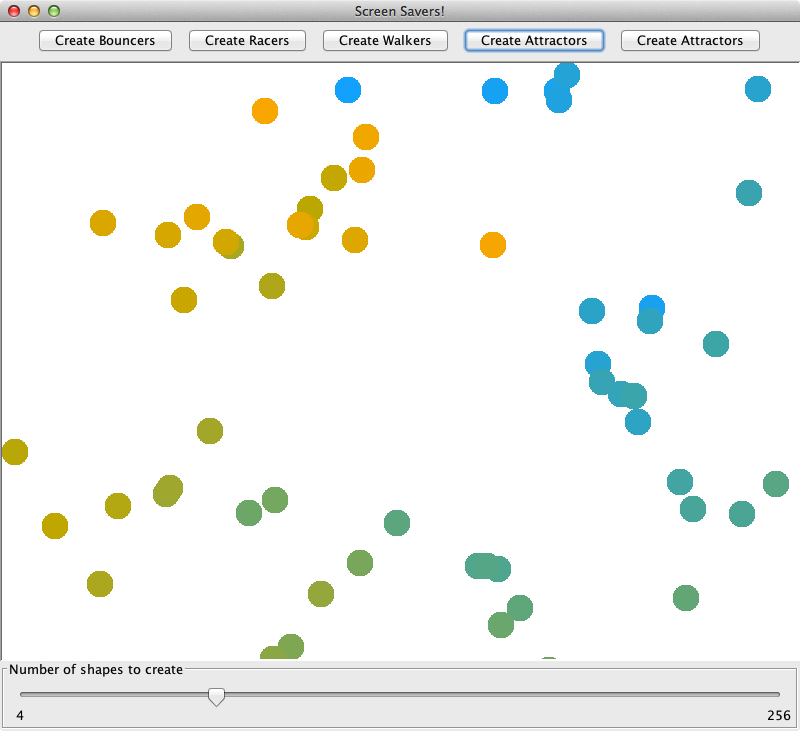
Testing
There are no JUnit tests required for this application (but you could write them for some classes/functionality), but you should make sure you test your application thoroughly so that you know it's working correctly. How can you do that?
Common Issues
- Placement of movers: Test with only 4 movers without any movement--that makes
the issues with placement clearer. You may also want to look at the
Mover
's methods too. - The objects don't extend the length/width of the screen. Why does that happen? How can you address that issue? (The solution is relatively simple.)
- The trail doesn't appear. Use print statements to see if you can determine the issue.
Submission
This isn't graded, but you should push your code into the GitHub repository.